How Well Have You Mastered Asyncio?
Gensen Huang
Created 6/26/2024
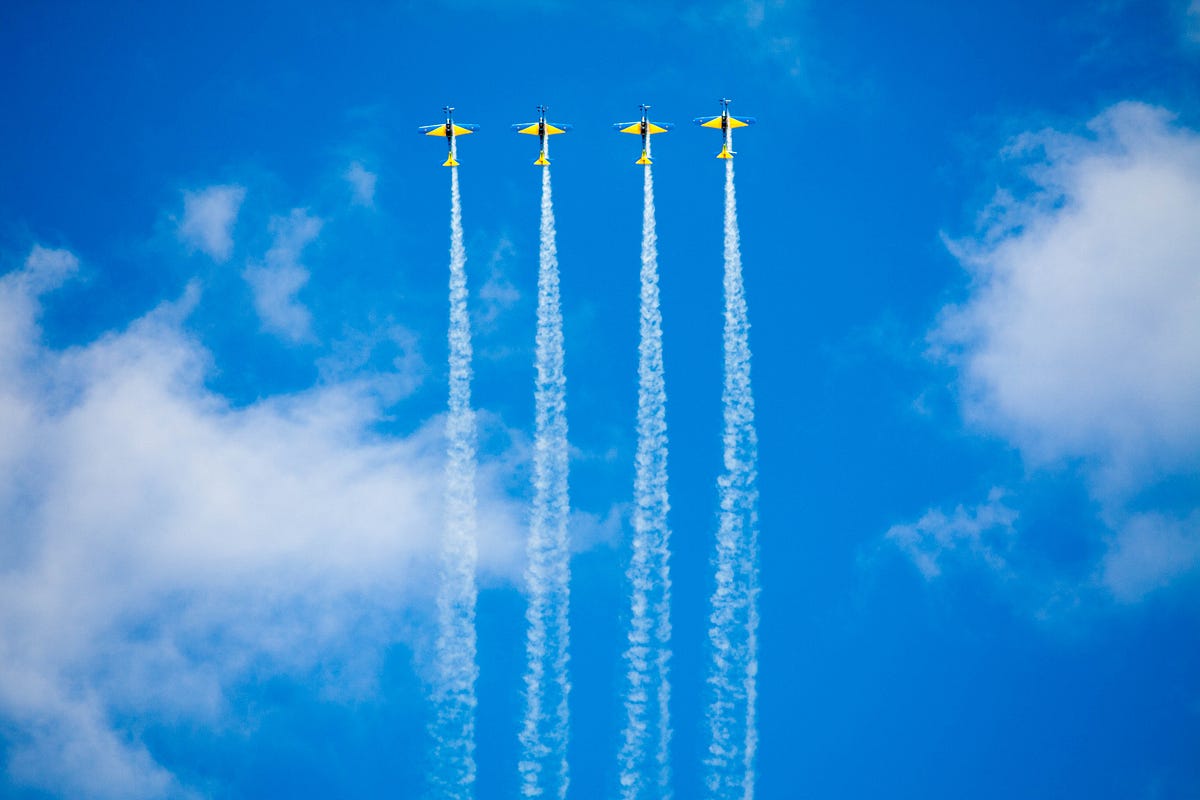
Test your knowledge of asyncio with this quiz and see how many correct answers you can get out of 15!
1. What is the main role of an event loop in asyncio?
To define coroutines
To manage synchronous tasks
To schedule and manage tasks, handle I/O operations and coordinate coroutines
To execute blocking operations
2. What keyword is used to define a coroutine in asyncio?
async def
await def
coroutine def
def async
3. Which function is used to run a coroutine in asyncio?
async.run()
asyncio.start()
await.run()
asyncio.run()
4. How do you pause a coroutine and allow other tasks to run?
use await keyword
use async keyword
use pause keyword
use yield keyword
5. What function is used to run multiple coroutines concurrently?
asyncio.run_all()
asyncio.concurrent()
asyncio.gather()
asyncio.parallel()
6. How do you create a task for a coroutine in asyncio?
asyncio.new_task()
asyncio.create_task()
asyncio.task()
asyncio.run_task()
7. What is the behavior of the asyncio.sleep() function?
Suspends a coroutine for a given number of seconds
Runs a coroutine immediately
Stops the event loop
Pauses the event loop indefinitely
8. What exception is raised if an asyncio task times out using asyncio.wait_for()?
asyncio.TimeoutError
asyncio.CancelledError
asyncio.TaskError
asyncio.TimeoutException
9. Which function is used to start an asyncio server?
asyncio.run_server()
asyncio.start_server()
asyncio.begin_server()
asyncio.serve()
10. Which library is commonly used with asyncio for HTTP clients and servers?
httpio
httplib
aiohttp
requests
11. What should you do to debug asyncio applications effectively?
Use asyncio.run() with debug=True
Set PYTHONASYNCIODEBUG environment variable to 1
Install asyncio debugger
Use traceback exception handler
12. What is the recommended Python version to use asyncio without needing a third-party library?
Python 2.7
Python 3.4
Python 3.6
Python 3.7 or higher
13. How do you handle exceptions in an asyncio coroutine?
Using try and catch blocks
Using try and except blocks
Using try and handle functions
Using try and error blocks
14. Which function is used to set a timeout for asynchronous operations?
asyncio.set_timeout()
asyncio.timeout()
asyncio.wait_timeout()
asyncio.wait_for()
15. How can you profile an asyncio application?
Using asyncio.profile()
Using Python’s cProfile module
Using asyncio's built-in profiler
Using the asyncio debug mode only